In this post, we'll walk through the process of setting up a Swagger UI for a Dart Shelf-backed application using the shelf_swagger_ui packageOur goal is to create a clean, modular implementation that follows best practices and is easy to maintain.
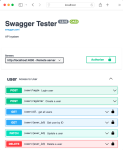
As shown in the screenshot above, we have a list of API endpoints related to the user module. These include common HTTP methods such as GET, POST, PATCH, and DELETE, which are used to reference specific actions in the API. Additionally, notice that the endpoints requiring authorization are marked with a lock icon, indicating that they require a bearer token for access.
Now, let's dive into the source code and see how we can implement this Swagger UI from scratch. Following the directory and file structure of project.
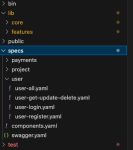
user-login.yaml
user-register.yaml
user-all.yaml
user-get-update-delete.yaml
user-components.yaml
And Finally swagger.yaml
Just Incase if Authorization is not availabe in your swagger-ui then add all the contents inside from
Please if there is any issues then please let me know in comment section below. Thank you.
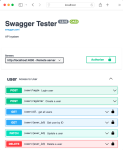
As shown in the screenshot above, we have a list of API endpoints related to the user module. These include common HTTP methods such as GET, POST, PATCH, and DELETE, which are used to reference specific actions in the API. Additionally, notice that the endpoints requiring authorization are marked with a lock icon, indicating that they require a bearer token for access.
Now, let's dive into the source code and see how we can implement this Swagger UI from scratch. Following the directory and file structure of project.
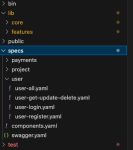
user-login.yaml
YAML:
post:
tags:
- "user"
summary: "Login user"
description: "Authenticates the user and returns user details if successful."
operationId: "login"
requestBody:
required: true
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
examples:
userLoginExample:
summary: "User login"
value: { "email": "[email protected]", "password": "password123" }
responses:
"200":
description: "Successful Operation"
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
description: "Bad Request - Invalid input"
content:
application/json:
schema:
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
YAML:
# /user/register:
post:
tags:
- "user"
summary: "Create a user"
requestBody:
required: true
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserRequestModel"
responses:
"200":
description: "successful operation"
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
Code:
# /user/all:
get:
tags:
- "user"
security:
- bearerAuth: []
summary: "get all users"
responses:
"200":
description: "successful operation"
content:
application/json:
schema:
type: array
items:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
YAML:
get:
tags:
- "user"
security:
- bearerAuth: []
summary: "Get user by ID"
parameters:
- in: path
name: user_id
required: true
schema:
type: string
minimum: 1
description: The user ID
responses:
"200":
description: "Successful operation"
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
patch:
tags:
- "user"
parameters:
- name: user_id
in: path
required: true
schema:
type: string
security:
- bearerAuth: []
summary: "Update a user"
requestBody:
required: true
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserRequestModel"
responses:
"200":
description: "Successful operation"
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
delete:
summary: "Delete a user"
parameters:
- name: user_id
in: path
required: true
schema:
type: string
tags:
- "user"
security:
- bearerAuth: []
responses:
"200":
description: "Successful operation"
content:
application/json:
schema:
$ref: "../components.yaml#/components/schemas/UserResponseModel"
"403":
$ref: '../components.yaml#/components/responses/Unauthorized'
"404":
$ref: '../components.yaml#/components/responses/NotFound'
"500":
$ref: '../components.yaml#/components/responses/InternalError'
YAML:
components:
securitySchemes:
bearerAuth:
type: http
scheme: bearer
bearerFormat: JWT # Optional, but helpful if you're using JWT tokens
description: "Auth header (Authorization) Access Token"
bearerAuthRefreshToken:
type: http
scheme: bearer
bearerFormat: JWT
description: "(Authorization) Refresh Token"
basicAuth:
type: http
scheme: basic
schemas:
UserRequestModel:
type: object
properties:
fullname:
type: string
phone:
type: string
isSocial:
type: boolean
username:
type: string
email:
type: string
password:
type: string
bio:
type: string
user_image:
type: string
UserResponseModel:
type: object
properties:
id:
type: string
fullname:
type: string
phone:
type: string
isSocial:
type: boolean
username:
type: string
email:
type: string
bio:
type: string
user_image:
type: string
created_at:
type: string
format: date-time
updated_at:
type: string
format: date-time
responses:
Unauthorized:
description: "Unauthorized"
content:
application/json:
schema:
type: object
properties:
message:
type: string
NotFound:
description: "Not Found"
content:
application/json:
schema:
type: object
properties:
message:
type: string
InternalError:
description: "Internal Server Error"
content:
application/json:
schema:
type: object
properties:
message:
type: string
# Global security requirement for all operations
security:
- bearerAuth: []
YAML:
openapi: 3.0.0
info:
description: "API system"
version: "1.0.10"
title: "Swagger Tester"
servers:
- url: http://localhost:4000
description: Remote server
tags:
- name: "user"
description: "Access to User"
- name: "project"
description: "Access to project"
paths:
/user/login:
$ref: "./user/user-login.yaml"
/user/register:
$ref: "./user/user-register.yaml"
/user/all:
$ref: "./user/user-all.yaml"
/user/{user_id}:
$ref: "./user/user-get-update-delete.yaml"
component.yaml
to end of swagger.yaml
file.Please if there is any issues then please let me know in comment section below. Thank you.