React with TypeScript gives us great tooling to write clean, reliable code.
In this post, we’ll look at how to pass props to components using objects, define types using interfaces, and make our code even cleaner using destructuring.
Let’s walk through it step by step.
Basic Component – No Props
This is a simple component that doesn’t take any props.
This just renders a heading and a button. No props involved yet.
Passing Props Using Object
Here we create a button component that takes a title prop as an object.
In this code:
- `MyButton` expects an object with one key: `title`, which is a string.
- We destructure it directly in the function parameter.
Multiple Props in an Object
Now let’s pass multiple props, for example, a title and a `disabled` flag.
Here we’re not using destructuring yet — we access props like `props.title` and `props.disabled`.
This is fine, but once the props list grows, it can get verbose.
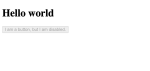
Defining Props with Interface
Instead of manually writing the object shape, we define a reusable type using an interface.
This improves readability and lets you reuse `MyButtonProps` elsewhere if needed.
Using Destructuring with Interface
Now let’s combine destructuring and interface — this is the cleanest approach.
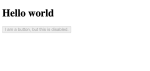
Here’s what we’re doing:
- `MyButtonProps` defines the shape of the props
- We destructure both `title` and `disabled` in the function parameter
- Code becomes shorter and more readable
Summary
- Use object typing for quick prop definitions
- Use interface for reusability and clarity
- Use destructuring for clean, concise code
This style is used a lot in real-world React + TypeScript projects. Try to get comfortable with interfaces and destructuring — it’ll make your code easier to read and maintain.
Here is the example with Using Union Types : react-using-union-types-in-props.20
Let me know if you want a version with event handlers or state too!
In this post, we’ll look at how to pass props to components using objects, define types using interfaces, and make our code even cleaner using destructuring.
Let’s walk through it step by step.

This is a simple component that doesn’t take any props.
JavaScript:
export default function MyApp() {
return (
<div>
<h1>Welcome to my app</h1>
<button>I'm Button</button>
</div>
);
}

Here we create a button component that takes a title prop as an object.
JavaScript:
function MyButton({ title }: { title: string }) {
return (
<button>{title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Hello world</h1>
<MyButton title="I'm a button" />
</div>
);
}
- `MyButton` expects an object with one key: `title`, which is a string.
- We destructure it directly in the function parameter.

Now let’s pass multiple props, for example, a title and a `disabled` flag.
JavaScript:
function MyButton(props: { title: string; disabled: boolean }) {
return (
<button disabled={props.disabled}>{props.title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Welcome to my app</h1>
<MyButton title="I'm a button, but I am disabled" disabled={true} />
</div>
);
}
This is fine, but once the props list grows, it can get verbose.
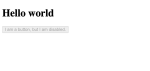

Instead of manually writing the object shape, we define a reusable type using an interface.
JavaScript:
interface MyButtonProps {
title: string;
disabled: boolean;
}
function MyButton(props: MyButtonProps) {
return (
<button disabled={props.disabled}>{props.title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Welcome to my app</h1>
<MyButton title="I'm a button, but I am disabled" disabled={true} />
</div>
);
}

Now let’s combine destructuring and interface — this is the cleanest approach.
JavaScript:
interface MyButtonProps {
title: string;
disabled: boolean;
}
function MyButton({ title, disabled }: MyButtonProps) {
return (
<button disabled={disabled}>{title}</button>
);
}
export default function MyApp() {
return (
<div>
<h1>Hello world</h1>
<MyButton title="I am a button, but this is disabled." disabled={true} />
</div>
);
}
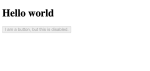
Here’s what we’re doing:
- `MyButtonProps` defines the shape of the props
- We destructure both `title` and `disabled` in the function parameter
- Code becomes shorter and more readable

- Use object typing for quick prop definitions
- Use interface for reusability and clarity
- Use destructuring for clean, concise code
This style is used a lot in real-world React + TypeScript projects. Try to get comfortable with interfaces and destructuring — it’ll make your code easier to read and maintain.
Here is the example with Using Union Types : react-using-union-types-in-props.20
Let me know if you want a version with event handlers or state too!
Last edited: