As a beginner, it's important to understand how to style HTML elements using CSS. Instead of writing all styles inside your HTML file, it’s a good practice to move the styles into a separate CSS file. This makes your code cleaner and easier to maintain.
In this guide, you’ll learn how to use class and id selectors in CSS, and how to apply those styles using an external CSS file.
1. HTML File (index.html)
We link the external stylesheet using a
2. CSS File (style.css)
Now let’s write all the CSS rules in a separate file called
3. Why Use External CSS?
- Keeps your HTML file clean
- Makes code reusable across multiple pages
- Easier to manage and debug styles
4. Summary Table
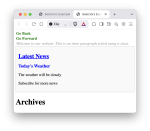
Conclusion
Now you know how to:
- Use class and ID selectors in HTML
- Move your CSS to an external file
- Apply structured and clean styling using real-world examples
Start organizing your HTML and CSS this way and you'll be writing clean, scalable code in no time!
In this guide, you’ll learn how to use class and id selectors in CSS, and how to apply those styles using an external CSS file.
1. HTML File (index.html)
We link the external stylesheet using a
<link>
tag inside the <head>
section.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Selectors Example</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<a class="navigation">Go Back</a>
<p class="navigation">Go Forward</p>
<p class="introduction">Welcome to our website. This is an intro paragraph styled using a class.</p>
<div id="blog">
<h1>Latest News</h1>
<div>
<h1>Today's Weather</h1>
<p>The weather will be cloudy</p>
</div>
<p>Subscribe for more news</p>
</div>
<div>
<h1>Archives</h1>
</div>
</body>
</html>
Now let’s write all the CSS rules in a separate file called
style.css
:
CSS:
/* Class selector for shared elements */
.navigation {
margin: 2px;
font-weight: bold;
color: green;
}
/* Specific class for paragraph */
p.introduction {
margin: 2px;
font-style: italic;
color: darkgray;
}
/* ID selector for the blog section */
#blog {
border: 1px solid #ccc;
padding: 10px;
background-color: #f9f9f9;
}
/* Targeting all h1 elements inside #blog */
#blog h1 {
color: blue;
}
/* Targeting nested h1 elements specifically */
#blog div h1 {
color: blue;
font-size: 18px;
}
/* Direct h1 child inside #blog only */
#blog > h1 {
font-size: 24px;
text-decoration: underline;
}
/* Hover effect for links */
a:hover {
color: orange;
cursor: pointer;
}
- Keeps your HTML file clean
- Makes code reusable across multiple pages
- Easier to manage and debug styles
4. Summary Table
Feature | Selector | Purpose |
Apply to multiple items | .class | Style repeated elements |
Apply to one unique item | #id | Style one specific section |
Make things interactive | :hover | Respond to user interaction |
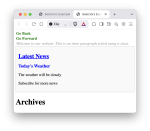
Conclusion
Now you know how to:
- Use class and ID selectors in HTML
- Move your CSS to an external file
- Apply structured and clean styling using real-world examples
Start organizing your HTML and CSS this way and you'll be writing clean, scalable code in no time!
Last edited: