Vite: A Modern Build Tool for React-TS Development
Vite is an amazing build tool that provides fast development and optimized builds for modern frontend frameworks. When you create a project using Vite with the React-TS template, it sets up everything for a smooth TypeScript-powered React development experience.
This guide explains the folder structure and files that come with `vite --template react-ts`, breaking them down level by level.
1. Project Initialization
To create a project, run:
Now, let’s dive into the structure!
2. Root Level Files
At the top level, you’ll see:
3. Inside src/ (Core of the Project)
This is where most of the development happens:
4. Understanding Key Files
main.tsx:
- This imports React and renders `<App />` into `#root`.
App.tsx:
- A basic React component with a button using state.
5. Vite Config (vite.config.ts)
- Uses Vite’s React plugin to enable JSX support.
6. TypeScript Configuration (tsconfig.json)
- Enforces TypeScript rules and module resolution.
7. Running the Project
To start the project:
Visit http://localhost:5173/ to see your app running!
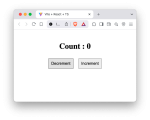
Conclusion
This setup ensures a fast and modern development experience with TypeScript support. With Vite, your project loads instantly, making development super efficient!
Vite is an amazing build tool that provides fast development and optimized builds for modern frontend frameworks. When you create a project using Vite with the React-TS template, it sets up everything for a smooth TypeScript-powered React development experience.
This guide explains the folder structure and files that come with `vite --template react-ts`, breaking them down level by level.
1. Project Initialization
To create a project, run:
Code:
npm create vite@latest my-app --template react-ts
cd my-app
npm install
npm run dev
2. Root Level Files
At the top level, you’ll see:
- package.json → Manages dependencies and scripts.
- vite.config.ts → Configures Vite’s behavior.
- tsconfig.json → TypeScript configuration.
- .eslintrc.cjs (optional) → ESLint rules for linting code.
- index.html → The main entry HTML file.
- public/ → Stores static assets like images.
3. Inside src/ (Core of the Project)
This is where most of the development happens:
- main.tsx → Entry file that mounts React to the DOM.
- App.tsx → The main React component.
- assets/ → Stores images, icons, etc.
- components/ → Contains reusable React components.
- styles/ (optional) → Global styles if used.
4. Understanding Key Files
main.tsx:
Code:
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
App.tsx:
Code:
import { useState } from 'react';
import './App.css';
function App() {
const [count, setCount] = useState(0);
return (
<div className="App">
<h1>Hello, Vite + React!</h1>
<button onClick={() => setCount(count + 1)}>
Clicks: {count}
</button>
</div>
);
}
export default App;
5. Vite Config (vite.config.ts)
Code:
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
export default defineConfig({
plugins: [react()],
});
6. TypeScript Configuration (tsconfig.json)
JSON:
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"jsx": "react-jsx",
"strict": true,
"baseUrl": ".",
"paths": {
"@components/*": ["./src/components/*"]
}
}
}
7. Running the Project
To start the project:
Code:
npm run dev
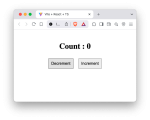
Conclusion
This setup ensures a fast and modern development experience with TypeScript support. With Vite, your project loads instantly, making development super efficient!
Last edited: